Developed based on Python API
Elephants provide Python APIs for remote control of robots, and we use TCP protocol to communicate between clients and robots. Therefore, before using our API, you need to follow the documentation to operate the following content.
1 Environment Building
1.1 Installing Python
Notice: Before installation, check the operation system of PC. Press right button on the
My Computer
icon and then selectProperties
. Install the corresponding Python.
- Go to http://www.python.org/download/ to download Python.
- Click on
Downloads
, and then download begins. TickAdd Python 3.10 to PATH
. Click onInstall Now
, and then installation begins.
Download and installation complete.
1.2 Running Python
Open the command prompt window (Win+R, input cmd
and press Enter
). Type Python
.
Successful Installation:
This on-screen instruction means that Python is successfully installed. The prompt >>>
means Python interactive environment. If you input a Python code to get the execution result immediately.
Error Report:
If a wrong instruction is typed, for example "pythonn", the system may report an error.
Notice: Generally, the error results from lack of environment configuration. Refer to 1.3 Environment Configuration to solve problems.
1.3 Environment Variable Configuration
Windows follows the path set by a Path environment variable in search of python.exe . Otherwise, an error will be reported. If you fail to tick Add Python 3.9 to PATH
during installation, you need to manually add the path where python.exe is located into environment variable or download python again. Remember to tick Add Python 3.9 to PATH
.
Follow the steps below to add python into environment variable manually.
- Right click on
My Computer
icon -->Properties ->Advanced System Settings ->Environment Variables
- The environment variables include user variables and system variables. For user variables, users can utilize their own downloaded programs via
cmd
command. Write the absolute path of the target program into the user variables.
- After the configuration, open the command prompt window (Win+R; input
cmd
and pressEnter
), and typePython
.
1.4 pymycobot installation
- pymycobot installation. Type
pip install pymycobot --upgrade --user
via terminal (Win+R)cmd
command.
pip install pymycobot --upgrade --user
2 Enable TCP server functionality
2.1 Log in to the RoboFlow operating system
After powering on the robot, use VNC Viewer to enter Raspberry Pi and log in to the RoboFlow operating system.
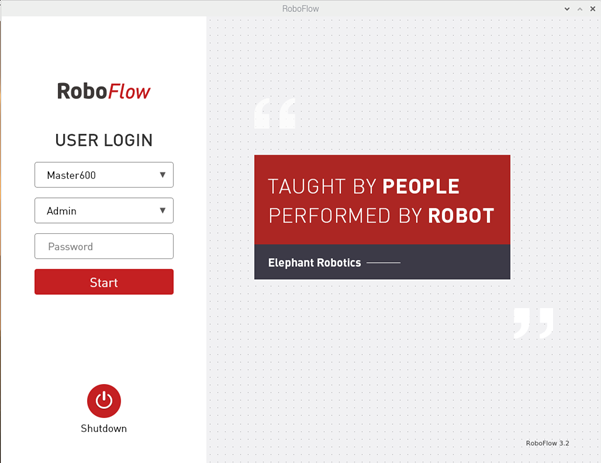
2.2 Start the robot
Enter the configuration center and click the start robot button.
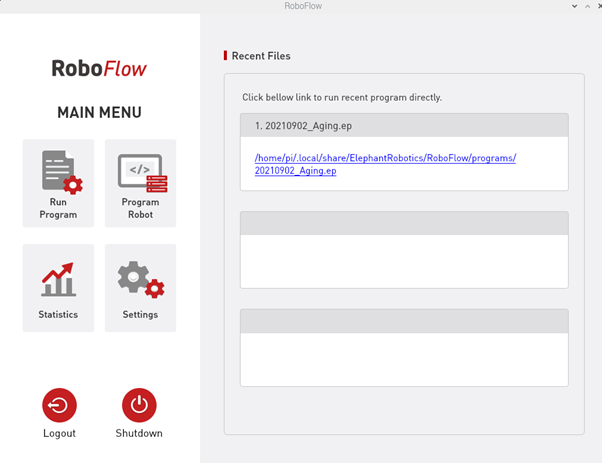
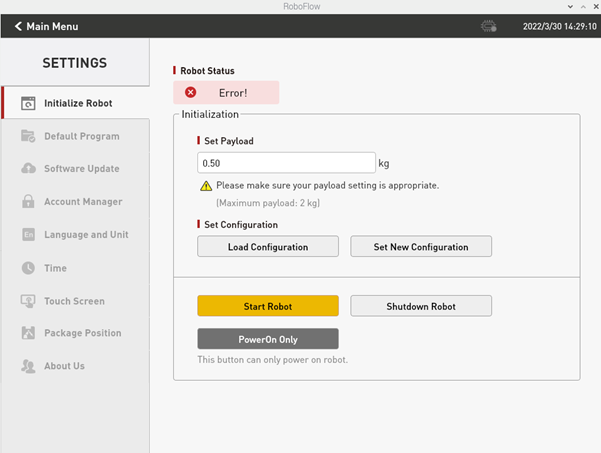
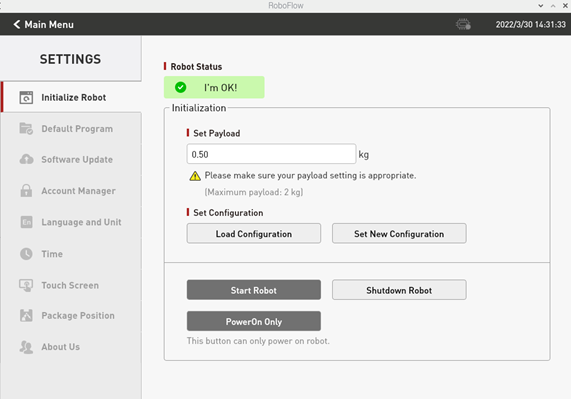
2.3 Check if the TCP server is enabled
Return to the main menu, click on Write Program, then click on Blank Program, enter the program editing interface, click on the Configuration button, click on the Network/Serial Port option, and check if the TCP server is enabled. Generally, the TCP server is enabled by default. If it is not enabled, it needs to be manually enabled.
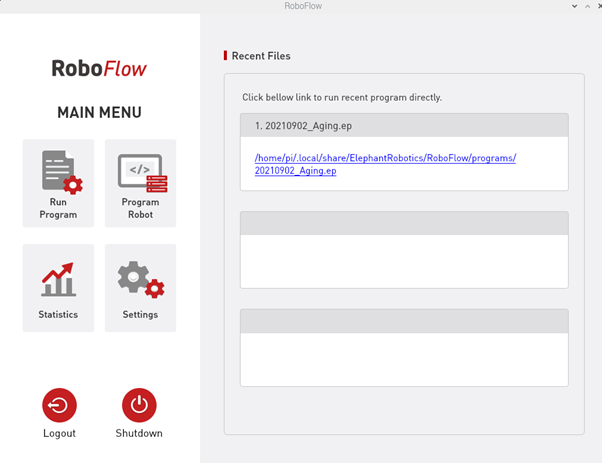
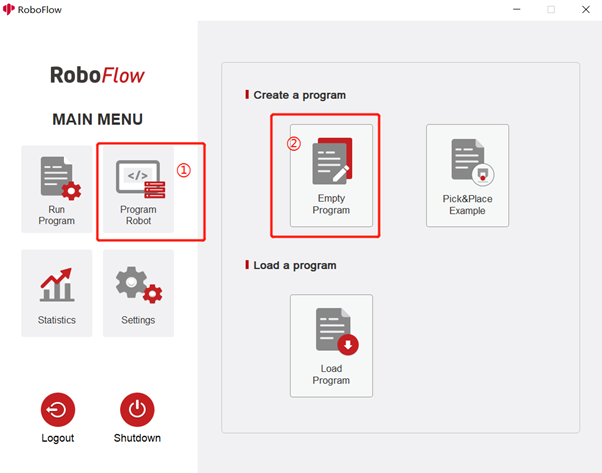
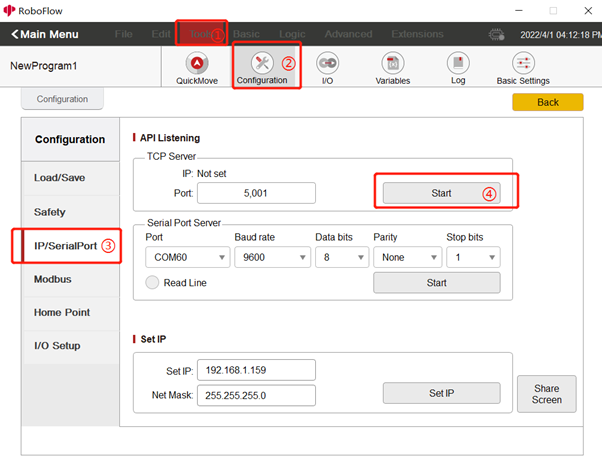
3 Introduction to API
3.1 ElephantRobot class instantiation
"Import ElephantRobot class from pymycobot library"
from pymycobot import ElephantRobot
"Connect to robot server"
elephant_client = ElephantRobot("192.168.137.182", 5001)
"Start TCP communication"
elephant_client.start_client()
To use the Python API, you must first instance the ElephantRobot class before calling the Function function of mycobot pro600
- Required Parameter:
- Parameter1: Actual IP address of the robot
Parameter2: Robot port (API function port fixed to 5001)
3.2 Introduction to Function Function
def start_client():
Function : Enable TCP connection ( To use Python API to control robots, this API must be called )
- Parameter : None
def stop_client():
- Function: Close TCP connection
- Parameter : None
def send_command(command):
- Function: Send instructions to the server
- Parameter : Instruction (string type)
def string_to_coords(data):
- Function: Convert string type data to list type data
- Parameter : String type data
def string_to_double(data):
- Function: Convert string type data to double precision floating-point data
- Parameter : String type data
def string_to_int(data):
- Function: Convert string type data to integer type data
- Parameter : String type data
def invalid_coords():
- Function: Return an invalid Cartesian coordinate to the server
- Parameter : None
def get_angles():
- Function: Requesting current joint angle information from the server
- Parameter : None
def get_coords():
- Function: Requesting current Cartesian pose information from the server
- Parameter : None
def get_speed():
- Function: Requesting robot motion rate from the server
- Parameter : None
def power_on():
- Function: Robot powered on
- Parameter : None
def power_off():
- Function: Robot power-off
- Parameter : None
def check_running():
- Function: Check if the robot is running
- Parameter : None
def state_check():
- Function: Get robot status
- Parameter : None
def read_next_error(data):
- Function: Robot error detection
- Parameter : None
def write_coords(coords,speed):
- Function: Send the overall coordinates and posture to move the mechanical alarm head from the original point to the specified point.
- Parameter: Cartesian pose of robot (list type), speed of mechanical alarm motion
def write_coord(axis, value, speed):
- Function: Send a single coordinate value to the robotic arm for movement ·
- Parameter: Cartesian position of the robot 0 represents x, 1 represents y, 2 represents, 3 represents r, 4 represents ry, 5 represents r2, the coordinate value to be reached, the speed of the mechanical alarm movement def write angles (angles, speed)
def write_angles(angles,speed):
- Function: Send all angles to all joints of the robotic arm
- Parameter: Joint angle (list type), speed of mechanical alarm movement
def write_angle(joint, value, speed):
- Function: Send the specified single joint motion to the specified angle
- Parameter: Specify joint [0 represents i1,1 represents i2,2 represents 3,3 represents 4,4 represents i5.5 represents i61, joint angle, mechanical alarm motion speed
def set_speed(percentage):
- Function: Set speed
- Parameter: Target speed
def set_carte_torque_limit(axis_str, value):
- Function: Set the torque limit of the robot
- Parameter: x/y/z/rx/ry/rz, twisted
def set_payload(payload):
- Function: Set the payload of the robot
- Parameter: Range 0.0-2.0
def state_on():
- Function: Starting the system
- Parameter : None
def state_off():
- Function: Shut down the system
- Parameter : None
def task_stop():
- Function: Task Pause
- Parameter : None
def jog_angle(joint_str, direction, speed):
- Function: Control the robot to continuously move at a specified angle .
- Parameter: The joints of the robotic arm [1/2/3/4/5/6] mainly control the direction of the machine's movement [1=negative direction, 0=stop, 1=positive direction], and the speed of the robot's movement
def jog_coord(axis_str, direction, speed):
- Function: Control the robot to continuously move in the specified coordinate axis direction
- Parameter: Cartesian direction [x//z/rx/ry/z], mainly controls the direction of machine alarm movement [-1=negative direction, 0=stop, 1=positive direction, the speed of robot movement
def get_digital_in(pin_number):
- Function: Obtain input pin signal
- Parameter: Pin number
def get_digital_out(pin_number):
- Function: Obtain output pin signal
- Parameter: Pin number
def set_digital_out(pin_number, pin_signal):
- Function: Set output pin signal
- Parameter: Pin number, pin status [0=low level, 1=high level]
def get_acceleration():
- Function: Obtain the acceleration of the robot
- Parameter : None
def set_acceleration(acceleration):
- Function: Set the acceleration of the robot
- Parameter: Acceleration
def command_wait_done():
- Function: Wait until the previous motion command is completed (this API function must be added after all motion API functions)
- Parameter : None
def wait(seconds):
- Function: Waiting time (in seconds)
- Parameter : None
def assign_variable(var_name, var_value):
- Function: Assign a value to a defined variable
- Parameter: Variable name (string type), target value
def get_variable(var_name):
- Function: Get the value of a variable
- Parameter: Variable name (string type)
4 Python API usage cases
4.1 Joint control
After using VNC Viewer to enter the RoboFlow system, in the fast moving interface, joint control can be used to control the robot to reach the target position and record the angles of the robot's six joints displayed on the operation panel.
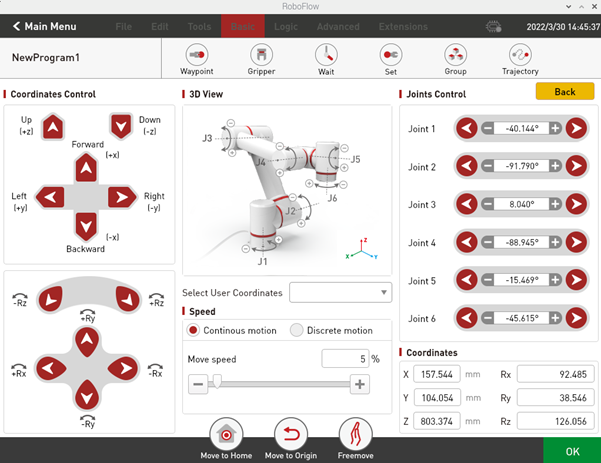
4.1.1 Multi joint control
from pymycobot import ElephantRobot
if __name__=='__main__':
"Connect to robot server"
elephant_client = ElephantRobot("192.168.137.182", 5001)
"Enable TCP communication"
elephant_client.start_client()
"Fill in the list of 6 recorded joint angles, with the last parameter being motion speed"
elephant_client.write_angles([94.828,-143.513,135.283,-82.969,-87.257,-44.033],1000)
"Wait for the robot to move to the target position before executing subsequent commands"
elephant_client.command_wait_done()
4.1.2 Single joint control
from pymycobot import ElephantRobot
if __name__=='__main__':
"Connect to robot server"
elephant_client = ElephantRobot("192.168.137.182", 5001)
"Enable TCP communication"
elephant_client.start_client()
"Fill in the angle of the individual joint to be controlled, with the first parameter 0 being the first axis, and so on; The second parameter represents the joint angle; The third parameter represents the speed of motion"
elephant_client.write_angle(0,94.828,1000)
"Wait for the robot to move to the target position before executing subsequent commands"
elephant_client.command_wait_done()
4.1.3 Joint angle acquisition
from pymycobot import ElephantRobot
if __name__=='__main__':
"Connect to robot server"
elephant_client = ElephantRobot("192.168.137.182", 5001)
"Enable TCP communication"
elephant_client.start_client()
"Print the current 6 joint angle information of the robot"
elephant_client.get_angles()
"Wait for the robot to move to the target position before executing subsequent commands"
elephant_client.command_wait_done()
4.2 Coordinate control
Mainly used to achieve intelligent route planning, allowing the robotic arm to move from one position to another specified position. Divided into [x, y, z, rx, ry, rz], where [x, y, z] represents the position of the robotic arm head in space (this coordinate system is a Cartesian coordinate system), and [rx, ry, rz] represents the attitude of the robotic arm head at that point (this coordinate system is an Euler coordinate system)
After entering the RoboFlow system using VNC Viewer, in the fast moving interface, the robot can be controlled through Cartesian coordinates. After reaching the target position, the robot's six coordinate values displayed on the operation panel can be recorded
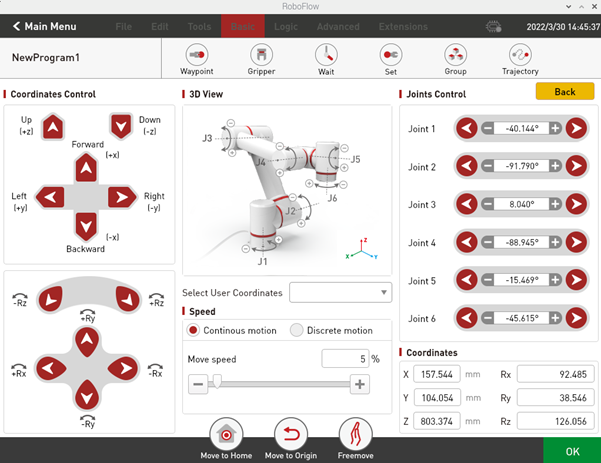
4.2.1 Multi parameter coordinate control
from pymycobot import ElephantRobot
if __name__=='__main__':
"Connect to robot server"
elephant_client = ElephantRobot("192.168.137.182", 5001)
"Enable TCP communication"
elephant_client.start_client()
"Fill in the list with the 6 coordinate values recorded, and the last parameter is the motion speed"
elephant_client.write_coords([-130.824,256.262,321.533,176.891,-0.774,-128.700], 3000)
"Wait for the robot to move to the target position before executing subsequent commands"
elephant_client.command_wait_done()
4.2.2 Single parameter coordinate control
from pymycobot import ElephantRobot
if __name__=='__main__':
"Connect to robot server"
elephant_client = ElephantRobot("192.168.137.182", 5001)
"Enable TCP communication"
elephant_client.start_client()
"Fill in the parameters, where the 2 of the first parameter represents the Z-axis direction, and so on; The second parameter represents the coordinate value of the relationship; The third parameter represents the speed of motion"
elephant_client.write_coord(2,94.828,3000)
"Wait for the robot to move to the target position before executing subsequent commands"
elephant_client.command_wait_done()
4.2.3 Cartesian space coordinate acquisition
from pymycobot import ElephantRobot
if __name__=='__main__':
"Connect to robot server"
elephant_client = ElephantRobot("192.168.137.182", 5001)
"Enable TCP communication"
elephant_client.start_client()
"Print out the current Cartesian space coordinate information of the robot"
elephant_client.get_coords()
"Wait for the robot to move to the target position before executing subsequent commands"
elephant_client.command_wait_done()
4.3 IO Control
4.3.1 Set IO pin output status
from pymycobot import ElephantRobot
if __name__=='__main__':
"Connect to robot server"
elephant_client = ElephantRobot("192.168.137.182", 5001)
"Enable TCP communication"
elephant_client.start_client()
"Control robot OUT1 output to high level"
elephant_client.set_digital_out(0,1)
"The robot will delay for 3 seconds before executing the subsequent program"
elephant_client.wait(3)
"Control robot OUT1 output to low level"
elephant_client.set_digital_out(0,0)
"The robot will delay for 3 seconds before executing the subsequent program"
elephant_client.wait(3)
4.3.2 Obtain IO pin output status
from pymycobot import ElephantRobot
if __name__=='__main__':
"Connect to robot server"
elephant_client = ElephantRobot("192.168.137.182", 5001)
"Enable TCP communication"
elephant_client.start_client()
"Obtain the output status of robot IN1"
elephant_client.get_digital_out(0)
"The robot will delay for 0.5 seconds before executing the subsequent program"
elephant_client.wait(0.5)
4.3.3 Set IO pin input status
from pymycobot import ElephantRobot
if __name__=='__main__':
"Connect to robot server"
elephant_client = ElephantRobot("192.168.137.182", 5001)
"Enable TCP communication"
elephant_client.start_client()
"Obtain the input status of robot IN1"
elephant_client.get_digital_in(0)
"The robot will delay for 0.5 seconds before executing the subsequent program"
elephant_client.wait(0.5)
5 Python API Application Scenario Cases
5.1 Handling and palletizing
from pymycobot import ElephantRobot
if __name__=='__main__':
"Connect to robot server"
elephant_client = ElephantRobot("192.168.137.182", 5001)
"Enable TCP communication"
elephant_client.start_client()
for i in range (1):
"Robot joints move to safety points"
elephant_client.write_angles([94.828,-143.513,135.283,-82.969,-87.257,-44.033],1000)
"Wait for the robot to move to the target position before executing subsequent commands"
elephant_client.command_wait_done()
"Transition point from Cartesian motion of robot to palletizing and grasping"
elephant_client.write_coords([-130.824,256.262,321.533,176.891,-0.774,-128.700], 3000)
elephant_client.command_wait_done()
"Control the robot to move Cartesian in the Z-axis direction to the stacking and grasping point"
elephant_client.write_coord(2,241.533,3000)
elephant_client.command_wait_done()
"Control robot OUT1 output to high level"
elephant_client.set_digital_out(0,1)
"Control the robot to wait for 1 second before taking action"
elephant_client.wait(1)
"Control the robot to move Cartesian in the Z-axis direction to the transition point for palletizing and grasping"
elephant_client.write_coord(2,321.533,3000)
elephant_client.command_wait_done()
"Control robot movement to placement transition point"
elephant_client.write_coords([86.687,255.542,320.867,177.065,-1.333,-128.721], 3000)
elephant_client.command_wait_done()
"Control the robot to move Cartesian in the Z-axis direction to the stacking placement point"
elephant_client.write_coord(2,241.533,3000)
elephant_client.command_wait_done()
"Control robot OUT1 output to low level"
elephant_client.set_digital_out(0,0)
elephant_client.wait(1)
"Control the robot to move Cartesian in the Z-axis direction to the transition point for stacking placement"
elephant_client.write_coord(2,321.533,3000)
elephant_client.command_wait_done()
"Robot joints move to safety points"
elephant_client.write_angles([94.828,-143.513,135.283,-82.969,-87.257,-44.033],1000)
elephant_client.command_wait_done()